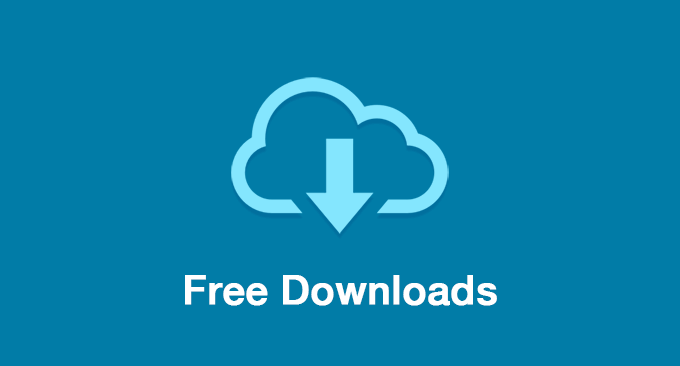
js下载文件
2022-08-02 16:36:35
2025-01-13 05:30:25
利用Blob对象
js
/**
* 下载文件
* @param {String} path - 下载地址/下载请求地址。
* @param {String} name - 下载文件的名字(考虑到兼容性问题,最好加上后缀名)
*/
import { ElMessage } from "element-plus";
export default {
downloadFile(path, name) {
const xhr = new XMLHttpRequest();
xhr.open("get", path);
xhr.responseType = "blob";
xhr.send();
xhr.onload = function () {
if (this.status === 200 || this.status === 304) {
const url = URL.createObjectURL(this.response);
const a = document.createElement("a");
a.style.display = "none";
a.href = url;
a.download = name;
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
URL.revokeObjectURL(url);
ElMessage.success("下载文件成功");
} else {
ElMessage.error("下载文件失败");
}
};
},
};
利用base64
js
/**
* 下载文件
* @param {String} path - 下载地址/下载请求地址。
* @param {String} name - 下载文件的名字(考虑到兼容性问题,最好加上后缀名)
*/
downloadFile (path, name) {
const xhr = new XMLHttpRequest();
xhr.open('get', path);
xhr.responseType = 'blob';
xhr.send();
xhr.onload = function () {
if (this.status === 200 || this.status === 304) {
const fileReader = new FileReader();
fileReader.readAsDataURL(this.response);
fileReader.onload = function () {
const a = document.createElement('a');
a.style.display = 'none';
a.href = this.result;
a.download = name;
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
};
}
};
}
目录