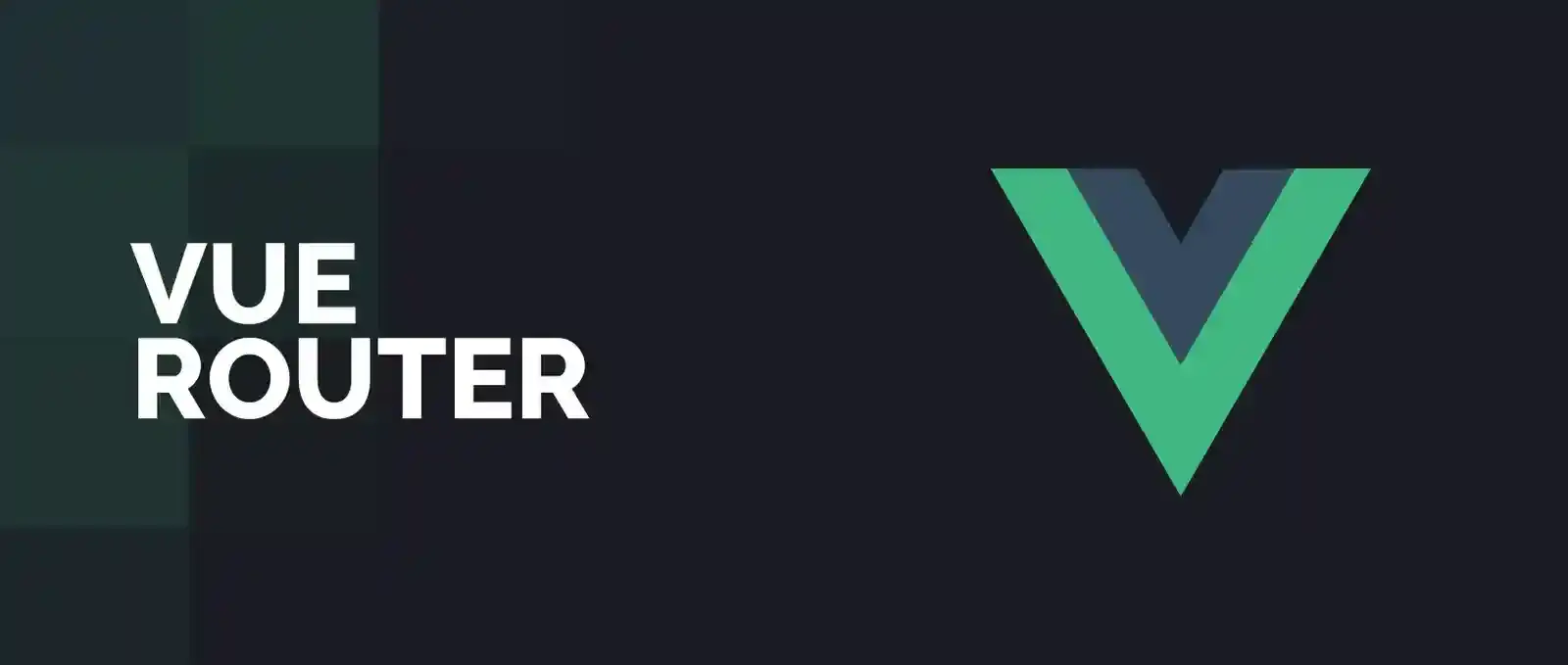
Vue-Router4入门到精通教程
2022-05-25 22:41:08
2025-04-26 03:18:52
为什么要使用 Vue-Router?
Vue Router 是 Vue.js 的官方路由。它与 Vue.js 核心深度集成,让用 Vue.js 构建单页应用变得轻而易举。
安装
shell
npm i -S vue-router@4
新建 src/router/index.ts
ts
import { createRouter, createWebHashHistory, RouteRecordRaw } from "vue-router";
export const asyncRoutes: Array<RouteRecordRaw> = [
{
path: "/",
//重定向到活动界面
redirect: "/activity",
meta:{
title:'首页',//配置title
keepAlive:false,//是否缓存
requireAuth:false//是否需要身份验证
}
},
//活动中心
{
path: "/activity",
name: "activity",
//路由懒加载
component: () => import("@/views/activity/Activity.vue"),
},
//通过ID进入藏品详细
{
path: "/collectionDetails/:id",
name: "collectionDetails",
component: () => import("@/views/collection-details/CollectionDetails.vue"),
},
];
const router = createRouter({
// 始终滚动到顶部
scrollBehavior() {
return { top: 0 };
},
history: createWebHashHistory(),
//设置路由前缀,默认为空
// history: createWebHashHistory("/cs"),
routes: asyncRoutes,
});
export default router;
在 main.ts 全局中使用
ts
import { createApp } from 'vue'
import router from "./router/index";
const app = createApp(App)
app.use(router)
app.mount('#app')
在 App.vue 全局中使用
vue
<template>
<div class="main">
<router-view></router-view>
</div>
</template>
核心概念与基本使用
history
在创建路由器实例时,
history
配置允许我们在不同的历史模式中进行选择。
模式 | 说明 | 区别 | Nginx 配置 |
---|---|---|---|
Hash | createWebHashHistory() | 有#,不需要服务端配合 | 无 |
HTML5 | createWebHistory() | 无#,需要服务端配合 | location / { try_files $uri $uri/ /index.html; } |
路由跳转
ts
import { useRouter } from "vue-router";
const router = useRouter();
//不带参数
router.push("/");
//路由query传参,参数拼接到url上,刷新不会丢失
router.push({
path: "/",
query: {
name:"小龙"
},
});
//路由params传参,注意params不能和path同时使用,要用params与name使用,刷新参数会丢失
router.push({
name: "/",
params: {
name:"小龙"
},
});
//路由params传参,注意params不能和path同时使用,要用params与name使用,刷新参数会丢失
router.push({
path: "/:name"
});
路由接受参数
ts
import { useRoute } from "vue-router";
const route = useRoute();
console.log(route.query.name);//小龙
console.log(route.params.name);//小龙
console.log(route.params.name);//小龙
- query 更加类似于 get 传参,在浏览器地址栏中显示参数,刷新不会丢失数据
- params 则类似于 post 传参,在浏览器地址栏中不显示参数,刷新会丢失数据
获取当前页面路由
route相当于当前正在跳转的路由对象 可以从里面获取name,path,params,query等
ts
import { useRoute } from "vue-router";
const route = useRoute();
console.log(window.location.href);//完整url
console.log(route.path);//路径
动态修改url,不刷新页面
vue-router修改
language
route.replace({path:'/name',query:{id:1}});
原生修改
参数 | 作用 |
---|---|
state | 状态对象是一个JavaScript对象,它与传递给 replaceState 方法的历史记录实体相关联. |
title | 大部分浏览器忽略这个参数, 将来可能有用. 在此处传递空字符串应该可以防止将来对方法的更改。或者,您可以为该状态传递简短标题 |
url | 历史记录实体的URL. 新的URL跟当前的URL必须是同源; 否则 replaceState 抛出一个异常. |
pushState
language
window.history.pushState(state, title[, url]) //添加并激活一个历史记录条目
js
// 如果当前的域名是 www.baidu.com
const state = { name: 'dx', age: 18 }
const title = ''
const url = '/about'
const result = history.pushState(state, title, url)
// 路由将会改变为www.baidu.com/about
replaceState
js
window.history.replaceState(state, title[, url]);
js
window.addEventListener('popstate', function (e) {
console.log(e.state)
},false)
const state1 = { name: 'dx', age: 18 }
const title = ''
const url = '/about'
history.pushState(state1, title, url)
history.back()
// 先后退到之前的路由,会打印一次state,但此时的路由没有通过pushState或者replaceState设置state,所以
// null
history.forward()
// 再次回到about路由时,又会打印一次state
// { name: 'dx', age: 18 }
虽然pushState和 replaceState改变路由时不能被popState监听到,但其它方式只要改变了浏览器路由,都会触发popState的监听,如果监听的路由恰好被pushState和replaceState设置过state,我们都能通过上面的方式拿到state。
重定向
ts
{
path: "/",
//访问'/',重定向到'/activity'
redirect: "/activity",
},
当没有匹配到正确路由的时候,重定向/404
ts
{
path: "/:pathMatch(.*)",
redirect: "/404",
},
导航守卫
通常用于检查用户是否有权限访问某个页面,验证动态路由参数,或者销毁监听器 自定义逻辑运行后,
next
回调用于确认路由、声明错误或重定向vue-router 4.x
中可以从钩子中返回一个值或Promise
来代替
ts
// to 将要访问的路径
// from 代表从哪个路径跳转而来
// next 是个函数,表示放行 next() 放行 next('/login') 跳转登录页面
router.beforeEach((to, from, next) => {
//设置浏览器标签页的标题
if (typeof to.meta.title === "string") {
document.title = to.meta.title;
}
// 如果用户访问的登录页,直接放行
if (to.path === "/login") return next();
//访问用户页面,兑换藏品
if (to.path === "/user") {
// 从 localStorage 中获取到保存的 token 值
const { token = "", idCardStatus } = storage.getItem("loginInfo") || {};
// 没有 token,强制跳转到登录页
if (!token) return next("/login");
//没有实名认证
if (idCardStatus === 0) return next("/realName");
next();
}
next();
});
动态路由
通常用于检查用户是否有权限访问某个页面,验证动态路由参数,或者销毁监听器 自定义逻辑运行后,
next
回调用于确认路由、声明错误或重定向vue-router 4.x
中可以从钩子中返回一个值或Promise
来代替
language
Routers.beforeEach((to,from,next) => {
if(to.path != '/' && !store.state.isLogin) {
//跳转的不是首页 同时 用户还未登陆
//这个判断,我们就可以基本判定用户是在做登陆时候的跳转
//↓拿到登陆时,接口返回的路由数组(vuex内),大致是这样的
let resRouterArr = [
{
routeName: '人员管理',
children: [
{
routeName: '销售管理',
...
},
{
routeName: '内勤管理',
...
}
]
}
]
let routerArr = []
resRouterArr.forEach(item => {
allRouters.forEach(all => {
if(item.routeName == all.routeName) {
//拿到本地路由对象
let obj = JSON.parse(JSON.stringify(all))
let childrenRouter = []
if(item.children && item.children.length > 0) {
item.children.forEach(childItem => {
all.children.forEach(allItem => {
if(childItem.routeName == allItem.routerName) {
childrenRouter.push(allItem)
}
})
})
obj.children = childrenRouter
}
routerArr.push(obj)
}
})
})
Routers.addroutes(routerArr)//addroutes为添加路由数组的方法
store.commit('domRouteTree',rousterArr)//存储进vuex,之后页面左右路由列表渲染使用
next({...to,replace:true})//进行路由跳转
} else {
next()
}
})
Vue Router 4: 路由参数在 created/setup 时不可用
Vue Router 4的破坏性变化是,现在所有的导航都是异步的,等待路由 ready 好后再挂载程序
language
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
const app = createApp(App)
app.use(router)
// Replace -> app.mount('#app')
router.isReady().then(() => {
app.mount('#app')
})
language
// 当参数更改时获取用户信息
watch(
() => route.params.id,
async newId => {
userData.value = await fetchUser(newId)
}
)
常见问题
目录